Introduction
There are circumstances that you don’t have an admin account on a machine and you need to install the latest Clang. Here, I explain how you can compile Clang 13 from the source on Ubuntu.
System
I use Ubuntu 20.04 installed on Windows 10 via Windows Subsystem for Linux (WSL).
GCC 9.3 was installed on Ubuntu.
Prerequisits
The system needs to have
- a C/C++ compiler like gcc/g++ and
- CMake alreay installed.
If you want to install GCC from source check this post.
Download
Go to the releases of LLVM on GitHub and get the URL of LLVM 13 (or the latest release) source code:
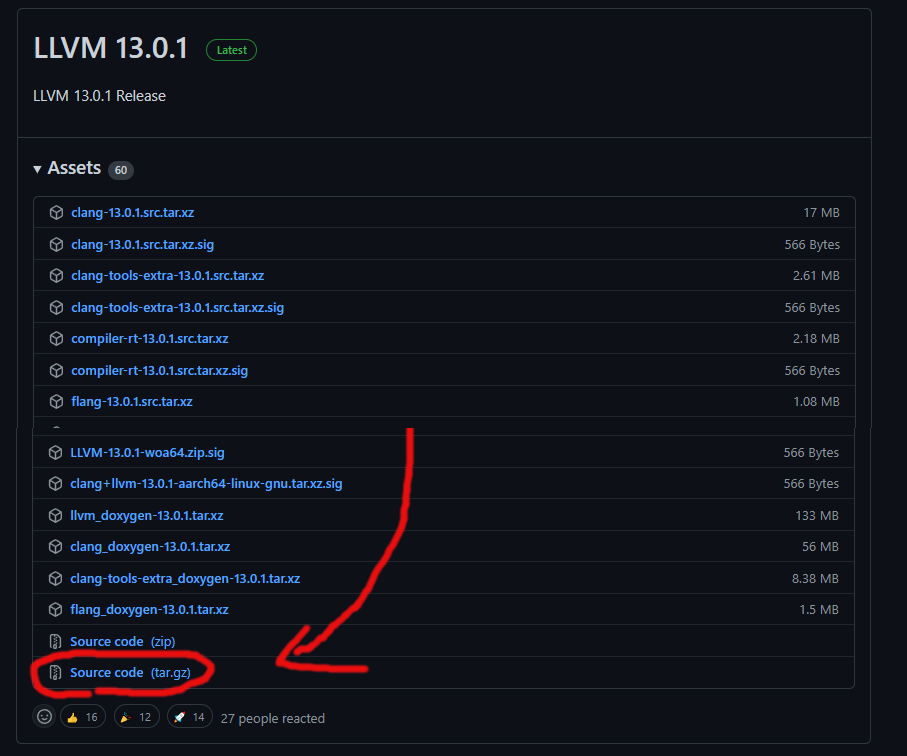
I assume you are installing the package in ~/apps
folder:
cd ~
mkdir apps
cd apps
wget URL_of_llvm_source
This downloads the source code. For me was llvmorg-13.01.tar.gz
, yours might be different name.
extract the compressed file via
tar -xvf llvmorg-13.01.tar.gz
This will extract the file in a folder named llvm-project-llvmorg-13.0.1
.
cd llvm-project-llvmorg-13.0.1
mkdir build
cd build
cmake -DLLVM_ENABLE_PROJECTS=clang -G "Unix Makefiles" ../llvm
make clang -j8
The last step compiles Clang with 8 CPU cores (my computer has 8 cores). The compilation took 30min for me.
All the executables are in the ~/apps/llvm-project-llvmorg-13.0.1/build/bin
folder including clang
(for C) and clang++
(for C++).
Now, you can compile a C++ test code this way
~/apps/llvmorg-13.01/build/bin/clang++ test.cpp
Add to path
To run executable from within any path, you can run the lines below in a terminal or add them to your ~/.bashrc
or ~/.bash_profile
:
clangbuild=~/apps/llvm-project-llvmorg-13.0.1/build
export PATH=$clangbuild/bin:$PATH
export LD_LIBRARY_PATH=$clangbuild/lib:$LD_LIBRARY_PATH
You can also create a file like load_clang13.sh
and paste the above lines in it. Then whenever you need Clang, you run
source load_clang13.sh
Test code
This code compiles with Clang 13+ because it supports the literal suffixes z
and uz
of C++23.
#include<iostream>
#include<type_traits>
int main(){
auto i = 1uz;
auto isSizet = std::is_same_v<decltype(i), std::size_t>;
std::cout<< std::boolalpha << isSizet <<"\n";
return 0;
}
It should compile with
clang++ test.cpp -std=c++2b
Latest Posts
- A C++ MPI code for 2D unstructured halo exchange
- Essential bash customizations: prompt, ls, aliases, and history date
- Script to copy a directory path in memory in Bash terminal
- What is the difference between .bashrc, .bash_profile, and .profile?
- A C++ MPI code for 2D arbitrary-thickness halo exchange with sparse blocks